How to upload Image in Node JS & Handle file size, file type error with Multer
upload Image in Node JS
Multer is a node.js middleware for handling multipart/form-data
, which is primarily used for uploading files. It is written on top of busboy for maximum efficiency.
NOTE: Multer will not process any form which is not multipart (multipart/form-data
).
Let’s add multer packge in our app. https://www.npmjs.com/package/multer
npm install multer --save
created a file > file-upload.js
const multer = require('multer'); const storage = multer.diskStorage({ destination: (req, file, callback) => { callback(null, './assets/avatar'); }, filename: (req, file, callback) => { callback(null, req.params.id + '_' + file.originalname); } }); let fileFilter = function (req, file, cb) { var allowedMimes = ['image/jpeg', 'image/pjpeg', 'image/png']; if (allowedMimes.includes(file.mimetype)) { cb(null, true); } else { cb({ success: false, message: 'Invalid file type. Only jpg, png image files are allowed.' }, false); } }; let obj = { storage: storage, limits: { fileSize: 200 * 1024 * 1024 }, fileFilter: fileFilter }; const upload = multer(obj).single('file'); // upload.single('file') exports.fileUpload = (req, res) => { upload(req, res, function (error) { if (error) { //instanceof multer.MulterError res.status(500); if (error.code == 'LIMIT_FILE_SIZE') { error.message = 'File Size is too large. Allowed file size is 200KB'; error.success = false; } return res.json(error); } else { if (!req.file) { res.status(500); res.json('file not found'); } res.status(200); res.json({ success: true, message: 'File uploaded successfully!' }); } }) };
Let’s understand code line by line…..
Multer Storage
The next thing will be to define a storage location for our files. Multer gives the option of storing files to disk, as shown below. Here, we set up a directory where all our files will be saved, and we’ll also give the files a new identifier.
We have created a folder assets & inside that created a folder named avatar.
We can update file name as per our requirement. Here I have put id and file original name.
destination: (req, file, callback) => { callback(null, './assets/avatar'); }, filename: (req, file, callback) => { callback(null, req.params.id + '_' + file.originalname); }
fileFilter
Set this to a function to control which files should be uploaded and which should be skipped. The function should look like this:
By below code we are checking file type. If coming file is not image file then simply return an error.
let fileFilter = function (req, file, cb) { var allowedMimes = ['image/jpeg', 'image/pjpeg', 'image/png']; if (allowedMimes.includes(file.mimetype)) { cb(null, true); } else { cb({ success: false, message: 'Invalid file type. Only jpg, png image files are allowed.' }, false); } };
limits
An object specifying the size limits of the following optional properties.
Now we have created a object where I have storage and limit of file size. In case coming file size is greater then defined size, multer will throw an error if file size.
let obj = { storage: storage, limits: { fileSize: 2 * 1024 * 1024 // 2 MB}, fileFilter: fileFilter };
Now we have to add our created object ‘obj’ into multer and called method single with param ‘file’. Here file is param of request body.
const upload = multer(obj).single('file');
Error handling
When encountering an error, Multer will delegate the error to Express. You can display a nice error page using the standard express way.
upload(req, res, function (error) { if (error) { //instanceof multer.MulterError res.status(500); if (error.code == 'LIMIT_FILE_SIZE') { error.message = 'File Size is too large. Allowed fil size is 200KB'; error.success = false; } return res.json(error); } else { if (!req.file) { res.status(500); res.json('file not found'); } res.status(200); res.json({ success: true, message: 'File uploaded successfully!' }); } })
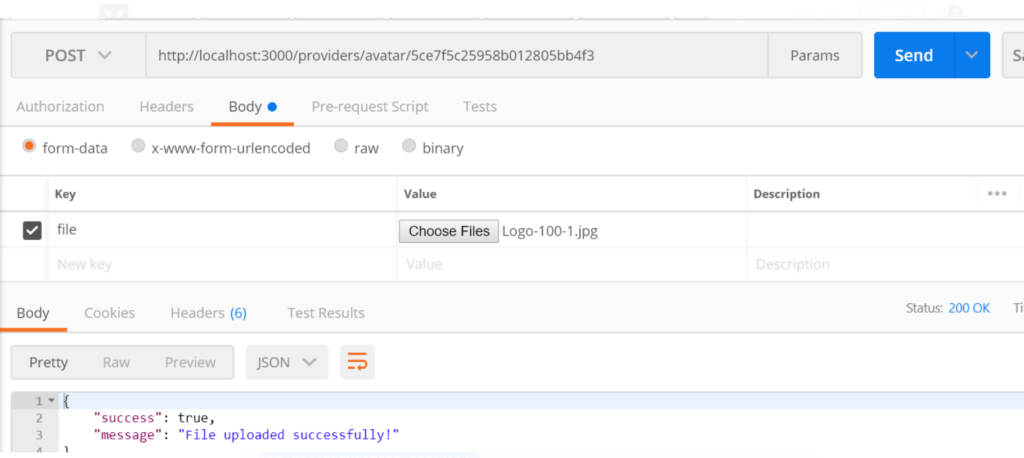
Run Application:
To run your application you can use any framework. Here I have used express.
const express = require('express');
const bodyParser = require('body-parser');
const port = 3000;
const app = express();
const router = express.Router();
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({extended: true}));
app.use('/static', express.static('assets'));
const router = express.Router();
const fileUploadCtrl = require('../controllers/file-upload');
router.post('/avatar/:id', fileUploadCtrl.fileUpload);
app.listen(port, () => console.log(app listening on port ${port}!
));
JS Mount https://jsmount.com
upload Image in Node JS…
WWW.M106.COM
Just discovered this blog through Bing, what a way to brighten up my day! Awesome article.