Frequently Asked HTML Interview Questions and Answers
HTML interview questions and answers, html interview questions for freshers, html interview questions jsmount, advanced html interview questions, top html interview questions, CSS and html interview questions, HTML 5 interview questions, Web development interview
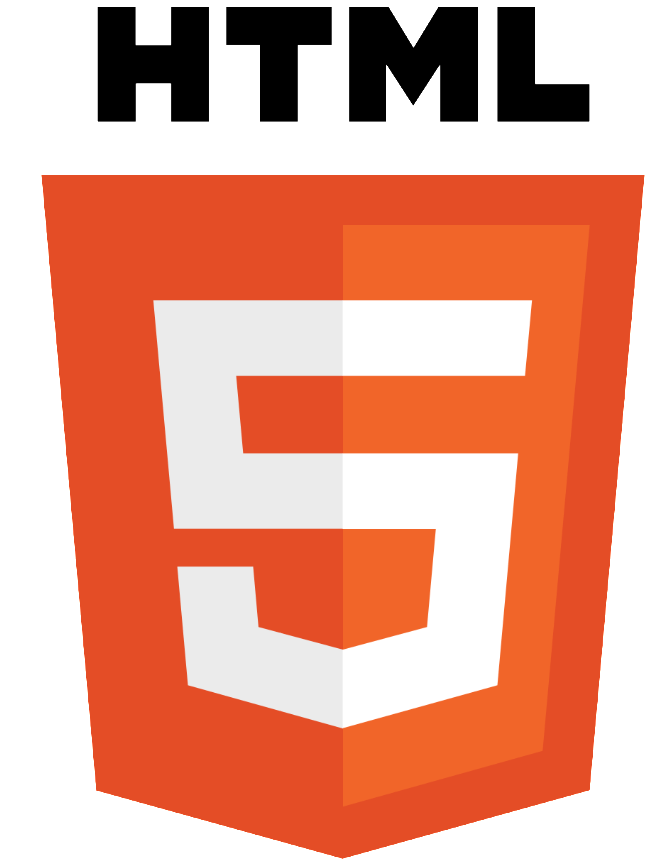
What is DocType in HTML?
First thing which we should know that it is not a HTML tag. It is an instruction to the browser about the version of the markup language the page is written in.
In HTML 5 we use doctype like <!DOCTYPE html>
How to vertically align text inside a flexbox?
We can do this by using display flex with some more properties:
justify-content: center; — center vertically.
flex-direction: column;
text-align: center; — to center horizontally.
li { display: flex; justify-content: center; flex-direction: column; text-align: center; }
What is Canvas in HTML 5?
Read here…
https://jsmount.com/html-create-canvas-graphics-with-javascript/
https://www.freecodecamp.org/news/full-overview-of-the-html-canvas-6354216fba8d/
What is Shadow DOM, Shadow Tree and Shadow Root?
http://robdodson.me/shadow-dom-the-basics/
What is the use of a Detail tag?
The tag specifies additional details that the user can view or hide on demand. Use it to create an interactive widget that the user can open and close. Semantically you can use any type of content inside it and should not be visible unless the open attribute is set.
<details open><p>This is test details<p></details>
How can we get all elements with the same class?
Element.getElementsByClassName() – This method returns an HTMLCollection that contains the same class given in the parameter.
How can we get all elements with the same tags?
Element.getElementsByTagName() – Returns HTMLCollection of elements that matches the tag passed in the parameter.
What is the difference between getElementByClassName vs getElementsByClassName?
getElementByClassName
return the first match node of passing a class in a parameter.getElementsByClassName
return all match elements as an HTML collection.
What is a query selector?
Element.querySelector() – It returns only the first node that matches the passed selector in the parameter.
Element.querySelectorAll() – It returns Node List.
What is the use of the “for” property with the label element?
By using for
attribute You can click the associated label to focus/activate the input, as well as the input itself. Many advantages of this to increase the hit area on the touch-screen devices.
<label for="username">User Name</label> <input type="text" name="username" id="username">
How do you change the number type in the middle of a list?
The <li>
tag includes two attributes – type and value
. The type attribute can be used to change the numbering type for any list item. The value attribute can change the number index.
Why should we use Semantic elements?
Semantic Elements gives meaning to the web browser rather than just
presentation. <h1> is an semantic element, so human knows what heading is and Browser knows how to display it. On another side, Nonsemantic elements do not provide any additional meaning to the markup.
What is empty elements?
Empty Elements are those elements that have no content. It does not have an end tag, such as the <br>
element.<br>
is an empty element without a closing tag (the tag is used to line break)
What is an image map in HTML?
The HTML <map>
element is used with <area>
elements to define an image map. we can click on that particular area and can move to any other links.
<img src="workplace.jpg" alt="Workplace" usemap="#workmap" width="400" height="379"> <map name="workmap"> <area shape="rect" coords="34,44,270,350" alt="Computer" href="computer.htm"> <area shape="rect" coords="290,172,333,250" alt="Phone" href="phone.htm"> <area shape="circle" coords="337,300,44" alt="Cup of coffee" href="coffee.htm"> </map>
Coords
is attribute of area tag
that is used with shape attributes. It tells the coordinate value of that area. It start from 0, 0 (top left corner area).
For more details read here…
https://www.w3schools.com/tags/att_area_coords.asp
How can we create a list with Roman number indexing?
We can do this with HTML Ordered List <ol> by using its type attribute.
type="1" for number sequence (default) type="A" for uppercase letters (A to Z) type="a" for lowercase letters (a to z) type="I" for uppercase roman numbers (I II III …) type="i" for lowercase roman numbers (i ii iii …) <ol type="I"> <li>India</li> <li>USA</li> </ol>
How can we change the counting start of the Ordered List?
We can do this with start
attributes.
<ol start="50"> <li>Apple</li> <li>Banana</li> <li>Milk</li> </ol>
What is the difference between session storage and local storage?
window.localStorage
– stores data with no expiration datewindow.sessionStorage
– stores data for one session (data is lost when the browser tab is closed)
What is the use of a blockquote tag?
The HTML Element indicates that the enclosed text is an extended quotation. A URL for the source of the quotation may be given using the cite attribute.
<blockquote cite="https://www.huxley.net/bnw/four.html"> <p>Words can be like X-rays, if you use them properly—they’ll go through anything. You read and you’re pierced.</p> <footer>—Aldous Huxley, <cite>Brave New World</cite></footer> </blockquote>
What are all the values of _target property?
The target attribute specifies where to open the linked document.
The target attribute can have one of the following values:
_blank - Opens the document in a new window or tab _self - Opens document in the same window/tab (default) _parent - Opens document in the parent frame _top - Opens document in the full body of the window framename - Opens the linked document in a named frame
How can I load Iframe when clicking on a link?
An iframe
can be used as the target frame for a link. The target attribute of the link must refer to the name attribute of the iframe:
<iframe src="a.html" name="myframe"></iframe> <p><a href="https://jsmount.com" target="myframe">JS Mount</a></p>
What is the use of the alt attribute of the IMG tag?
The alt attribute is used as an alternate text for an image. It displays in case the image does not load or any internet connection issues.
What is difference between Absolute File Paths & Relative File path?
An absolute file path is a full URL to an internet file:<img src="https://www.abc.com/images/demoimage.jpg" alt="Demo Image">
A relative file path points to a file relative to the current page.<img src="/images/abcd.jpg" alt="Profile Picture">
How can we restrict file type in the input type file field?
accept: A string that defines the file types the file input should accept.
<input type="file" id="avatar" name="avatar" accept="image/png, image/jpeg">
Do you know the input type telephone, Url?
The <input type="tel">
is used for input fields that should contain a telephone number. <input type="tel" name="phone" pattern="[0-9]{3}-[0-9]{2}-[0-9]{3}"> It will take format like 123-45-678.
The <input type="url">
is used for input fields that should contain a URL address. the URL field can be automatically validated when submitted.
What is GeoLocation Api of HTML?
The HTML Geolocation API is used to locate a user’s position.
getCurrentPosition() method is used to get current position of User. if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function() {}); }
Which are important methods used to drag & drop data in HTML?
function drag(ev) { ev.dataTransfer.setData("text", ev.target.id); } The dataTransfer.setData() method sets the data type and the value of the dragged data. function drop(ev) { ev.preventDefault(); var data = ev.dataTransfer.getData("text"); ev.target.appendChild(document.getElementById(data)); }
Call preventDefault() to prevent the browser default handling of the data (default is open as a link on the drop) Get the dragged data with the dataTransfer.getData()
method. This method will return any data that was set to the same type in the setData() method The dragged data is the id of the dragged element (“drag1”) Append the dragged element into the drop element
html interview questions and answers, html interview questions for freshers, html interview questions jsmount, advanced html interview questions, top html interview questions, CSS and html interview questions, HTML 5 interview questions, Web development interview
- Unlocking the Secrets of Cookie | Power Your Web Experience
- AI vs Human: Exploring the Boundaries of Intelligence
- Enhancing User Experience with JavaScript Throttle and Debounce
- JavaScript Custom Promise | Promise Polyfill
- JavaScript Promisify | Convert Callbacks to Promises
- JavaScript Promise and Callbacks | Everything You Must Know
- CSS Display Property – Deep dive in block | inline | inline-block
- Implementation of Queue using Linked List | JavaScript
- Insert node in Linked list | Algorithm | JavaScript
- Insertion Sort in data structure | Algorithm with Examples
- Selection Sort Algorithm & K’th Largest Element in Array
- Quick Sort Algorithm with example | Step-by-Step Guide
- Dependency Inversion Principle with Example | Solid Principles
- Object-Oriented Programming | Solid Principles with Examples
- ASCII Code of Characters | String Operations with ASCII Code
- Negative Binary Numbers & 2’s Complement | Easy explanation
- Factors of a Number | JavaScript Program | Optimized Way
- LeetCode – Game of Life Problem | Solution with JavaScript
- Fibonacci series using Recursion | While loop | ES6 Generator
- JavaScript Coding Interview Question & Answers
- LeetCode – Coin Change Problem | Dynamic Programming | JavaScript
- HackerRank Dictionaries and Maps Problem | Solution with JavaScript
- React Redux Unit Testing of Actions, Reducers, Middleware & Store
- Micro frontends with Module Federation in React Application
- React Interview Question & Answers – Mastering React
- Top React Interview Question & Answer | React Routing
- React Interview Questions and Answers | React hooks
- Higher Order Component with Functional Component | React JS
- Top React Interview Questions and Answers | Must Know
- Interview Question React | Basics for Freshers and Seniors
- Cyber Security Fundamental Questions & Answers You must know
- Application & Web Security Interview Questions & Answers
- Top Scrum Master and Agile Question & Answers 2022
- Trapping Rain Water Leetcode Problem Solution
- Array Representation of Binary Tree | Full Tree & Complete Binary Tree
- Graphs in Data Structure, Types & Traversal with BFS and DFS, Algorithms
- Traversing 2 D array with BFS & DFS Algorithm in JavaScript
- Time Complexity & Calculations | All You should know
- Backspace String Compare Leetcode Problem & Solution
- Angular Interview Questions & Answers 2021 – Part 3 – JS Mount
- Why Angular is a Preferred Choice for Developers? Top Features
- Angular Interview Questions & Answers You should know Part 2
- Top 30 JavaScript Interview Questions and Answers for 2021
- React JS Stripe Payment Gateway Integration with Node | Step by Step Guide
- Create Year Month & Date dropdown List using JavaScript & JQuery
- Create Custom QR Code Component using QR Code Styling in React JS
- How to create a common Helper class or util file in React JS
- React Build Routing with Fixed Header and Navigation | React Router Tutorial
- React Create Dashboard Layout with Side Menu, Header & Content Area
- Web Application Security Best Practices | Top Tips to Secure Angular App
Von Lawing
Excellent blog post. I certainly love this website. Stick with it!|
Tennille Ternes
It’s a shame you don’t have a donate button! I’d without a doubt donate to this brilliant blog! I suppose for now i’ll settle for bookmarking and adding your RSS feed to my Google account. I look forward to new updates and will talk about this site with my Facebook group. Chat soon!|
더킹 사이트
Thanks for sharing your info. I truly appreciate your efforts and I will be waiting for your further write ups thanks
once again.
Carolyne Stoffey
I read this article completely regarding the difference between the newest and earlier technologies, it’s an awesome article.|
เว็บพนัน
I do not even know how I ended up here, but I thought this post was good. I do not know who you are but definitely, you’re going
to a famous blogger if you are not already 😉 Cheers!
sekolahbisnisonlineindonesia.com
Wow! Finally, I got a web site from where I will be capable of genuinely obtain valuable data regarding my study and knowledge.
바카라사이트
Greetings! Very useful advice in this particular article!
It is the little changes that will make the biggest changes.
Many thanks for sharing!
바카라사이트
Hi, I do think this is an excellent blog. I stumbled upon it 😉 I am going to come back yet again since i have bookmarked it.
Money and freedom is the best way to change, may you be rich and continue to help
other people.
sddcmaster.com
you’re really a just right webmaster. The site loading velocity is amazing.
It kind of feels that you’re doing any distinctive trick.
Moreover, The contents are masterpiece. you’ve performed a fantastic task
in this matter!
merissa
Way cool! Some extremely valid points! I appreciate you writing this article
plus the rest of the website is extremely good.
marc_boose
I got this website from my friend who informed me concerning this website
and at the moment this time I am visiting this site and reading very informative articles at this place.
roslyn
Hello, just wanted to tell you, I enjoyed this blog post.
It was inspiring. Keep on posting!
더킹카지노
I think this is one of the most significant info for me. And I’m glad to read your article.
But want to remark on some general things, The site style is ideal,
the articles are really excellent: D. Good job, cheers
우리카지노
I read this post fully about the resemblance of latest and previous technologies,
it’s an amazing article.
Andy Bronchetti
Many thanks for sharing this great write-up. Very inspiring! (as always, btw)
check out the post right here
I found your blog website on google as well as inspect a few of your early articles. Remain to keep up the very good run. I simply added up your RSS feed to my MSN Information Visitor. Seeking forward to learning more from you later!?
John Deere Repair Manuals
you employ a fantastic weblog here! want to earn some invite posts on my blog?
cannabidol
I like this website it’s a master piece! Glad I found this on google.
Torsten
That is a great tip especially to those fresh to the blogosphere.
Brief but very accurate information… Many thanks for sharing this one.
A must read article!
Luann Harrier
Bookmarked!, I love your website!