JavaScript Console Interview Questions | Input Output Program
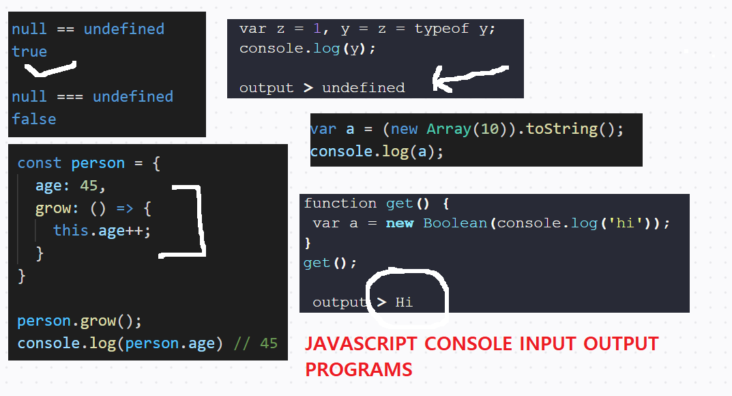
JavaScript Console Interview Questions
Write a program that can produce the same result by below two ways.
console.log(sum(2, 3)); console.log(sum(2)(3)); function sum(a, b) { function innerSum(b) { return a + b; } if (a && b) { return innerSum(b); } else { return innerSum; } }
Shift all Negative numbers of the array at starting and positive numbers after that in the array.
Example :
var x =[15,-4, 10, 12, -12, 10, -11, 23, 8372]; output should be :
output is > [-11, -12, -4, 15, 10, 12, 10, 23, 8372]
for (let i = 0; i < x.length;i++){ if(x[i] < 0) { x.unshift(x.splice(i, 1)[0]); } } console.log(x);
What is the output of the below code?
var a; var b = (a = 3)? true : false; console.log(a, b); // output > 3 true
console.log(sum(10,20)); console.log(diff(10, 20)); function sum(x, y) { return x + y; } let diff = function(x, y) { return x - y; } // output 30 ReferenceError: Cannot access 'diff' before initialization
class X { z = 10; get Y() { return 40; } } var x = new X(); console.log(x.Y()) // TypeError: x.Y is not a function console.log(x.Y, x.z) // 40 10
var v = 1; var fun1 = function() { console.log(v); } var fun2 = function() { var v = 2; fun1(); } fun2(); // output -> 1
(function(){ var a = b = 3; })(); console.log(typeof a ! == ‘undefined’);
The answer is false. Explanation is : typeof anythingyoucantypehere is ‘undefined’
var a = (new Array(10)).toString(); console.log(a); output > ",,,,,,,,,"
Number('1') == 1; output > true
const human = { name: 'Mohit', age: 25 } human.age = 26; console.log(human.age) // 26
const person = { age: 45, grow: () => { this.age++; } } person.grow(); // Inside grow, this is an window object console.log(person.age) // 45 const person1 = { age: 45, grow: function() { this.age++; } } person1.grow(); console.log(person1.age) // 46
function hello() { var a = 10; function One() { a = 11; } function Two() { return a; } Two(); } console.log('Print hello', hello()); // return statement is in inner function Two, not from main function. output > 'Print hello' undefined
function fun(input) { var r = 12; if (input) { let t = r + 1; return t; } return t; } console.log(fun(true)); // 13 console.log(fun(false)); // ReferenceError: t is not defined
function fun1() { if (true) { let q = w = 1; } console.log(w); // 1 console.log(q); // ReferenceError: q is not defined } // Here w is defined as global variable and q is local variable fun1();
What is the output of JavaScript Math inbuilt apis?
- The
Math.round()
function returns the value of a number rounded to the nearest integer. - The
Math.floor()
function returns the largest integer less than or equal to a given number. - The
Math.ceil()
function always rounds a number up to the next largest integer.
Math.round(11.49) 11 Math.round(11.51) 12 Math.floor(11.49) 11 Math.floor(11.51) 11 Math.ceil(11.49) 12 Math.ceil(11.51) 12
What is the output?
function parent() { var hoisted = "I am a variable"; function hoisted() { return "I’m a function"; } return hoisted(); } parent();
output is > Uncaught TypeError: hoisted is not a function
Explanation :
Looks below code, it gives a better idea. After each line, we have added console.log
function parent() { console.log("1", hoisted); var hoisted = "I’m a variable"; console.log("2", hoisted); function hoisted() { return "I'm a function"; } console.log("3", hoisted); } parent();
output>
1 ƒ hoisted() { return “I’m a function”; } 2 I’m a variable 3 I’m a variable
What is the output?
var y = 1; if (function f(){}) { y += typeof f; } output > “1object”
null == undefined output > true null === undefined output > false
What will be output?
var y = 1; if ( void function f(){}) { y += typeof f; console.log(‘1’, y); } console.log(‘2’, y); output > 2 1 // first console.log will not run because of expression inside if condition give undefined and that is equal to false so if condition will not be executed for this scenario.
What is the output?
void function employee(){ console.log(‘my name’); } employee(); output > Uncaught Reference Error: the employee is not defined // because if we put any valid expression after void, it always gives undefined.
What is the output of the below code? Nice Question !!!
console.log(‘a’) && console.log(‘b’); console.log(‘a’) || console.log(‘b’); console.log('a' && 'b'); console.log('a' ||'b'); output > a a b b a
Explanation:
First expression is bind with the AND (&&) operator so the only first condition will be executed because console.log(‘a’) return undefined and that is equal to false. And in && condition, if the first statement is false, second does not run.
In the Second expression it is bind with OR (||) so when first return undefined, it checks the second statement.
What is the output?
function emp() { var x = 10; return emp; } function emp() { var x = 10; return emp; function emp(name) { return name; } } Output > First function will return the same emp function body. And the second function will return the body of inner emp function instead of parent one because of hoisting concept of JS
What is the output?
const arr = [10, 12, 15, 21]; for (var i = 0; i < arr.length; i++) { setTimeout(function() { console.log('Index: ' + i + ', element: ' + arr[i]); }, 3000); } output > 4 times it will print Index: 4, element: undefined
What is the output?
if("2 < 4") { console.log('hi'); } if("2 > 4") { console.log('hi'); } output > Hi Hi
What is the output?
typeof hhhhhhhhhhhh "undefined" typeof String "function" typeof Boolean "function" typeof string "undefined" typeof boolean "undefined"
console.log({} == true); console.log([] == true); if({}) {console.log('works')}; if([]) {console.log('works')}; output > false false works works
console.log(new String({})); console.log(new String([])); output > String {“[object Object]”} String {“”, length: 0}
if(NaN == NaN) {console.log('hi')}; output > nothing
var b = new Boolean(false); if(b) {console.log('hi')}; output > Hi
let x; true || (x = 1); alert(x); output > undefined
function get() { var a = new Boolean(console.log('hi')); } get(); output > Hi
new Boolean([]); new Boolean([].length); output > Boolean {true} Boolean {false}
var arr = [1,2,3]; console.log(arr instanceof Array, arr instanceof Object); Output > true true var obj = {a: 12}; console.log(obj instanceof object); output > ReferenceError: object is not defined var obj = {a: 12}; console.log(obj instanceof Object); output > true
What is the best way to find which one is an array and which one is an object?
var a = [1,2,3]; var b = {a : 23}; One way > Object.prototype.toString.call(a) === "[object Object]" Object.prototype.toString.call(b) === "[object Array]" Second Way > a instanceof Array => true b instanceof Array => false Third way > a.length => defined b.length => not defined
How many ways can we create a duplicate of an array?
const names = [ 'Jon', 'Jacob', 'Jeff' ] Most Used way > const copy1 = [...names]; const copy2 = Object.assign([], names); const copy3 = JSON.parse(JSON.stringify(names)); // create deep copy const copy4 = names.slice(); Other way > const copy2 = [].concat(names); const copy3 = Object.values(names); const copy5 = Array.of(...names); const copy7 = names.map(i => i);
What is the output?
var z = 1, y = z = typeof y; console.log(y); output > undefined
var arr = [a = 1, b = 2, 3, 4]; console.log(arr, b); output > [1,2,3,4] 2; var arr = [1 == 1, b = 2, 3, 4]; console.log(arr); output > [true, 2,3,4]
(function emp() { (function cust() { var a = 0; return (a = 1 + 4); })(); a = 6; return a; })(); output > 6
const [a, b, c, d] = (function emp() { return [1, 2, 3, 4]; })(); console.log(b, d); output > 2 4
function find(arr) { arr.forEach((item) => { if (item == 1) { return item; } }); } find([1,2]); output > undefined // we cannot return direct from for-each method of array. ***Correct Way to do this*** function find(arr){ let output = ''; arr.forEach(item =>{ if(item == 1) { return output = item; } }); return output; } find([1,2]);
x = y; y = 9; console.log(x,y); output > Uncaught Reference Error: y is not defined x= y; var y = 9; console.log(x,y); output > undefined 9
function check(x,y,z){ return (x,y,z); } check(1,2,3) output > 3 // when we return data by comma separated , it returns last variable. function check(x = 1, y = x) {return (x,y);} check(); output > 1 function check(x = y, y = 3) {return (y, x);} check(); output > y is not defined function check(x = y, y = 3) {return x;} check(); output > y is not defined
var a = undefined; console.log(a.length); output > Cannot read property 'length' of undefined var b = 123456; console.log(b.length); output > undefined var c = null; console.log(c.length); output > Cannot read property 'length' of null
JavaScript Console Interview Questions
HTML advance Interview Questions and Answers for Experienced – Part 1
4 thoughts on “JavaScript Console Interview Questions | Input Output Program”